04. Model View Controller (MVC)
Solution Code to the previous section
todoapp/app.py
from flask import Flask, render_template
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'postgres://udacitystudios@localhost:5432/todoapp'
db = SQLAlchemy(app)
class Todo(db.Model):
__tablename__ = 'todos'
id = db.Column(db.Integer, primary_key=True)
description = db.Column(db.String(), nullable=False)
def __repr__(self):
return f'<Todo {self.id} {self.description}>'
db.create_all()
@app.route('/')
def index():
return render_template('index.html', data=Todo.query.all())
todoapp/templates/index.html
<html>
<head>
<title>Todo App</title>
</head>
<body>
<ul>
{% for d in data %}
<li>{{ d.description }}</li>
{% endfor %}
</ul>
</body>
</html>
terminal commands executed
$ create todoapp
$ FLASK_APP=app.py FLASK_DEBUG=true flask run
psql todoapp
>>> \dt
>>> \d todos
>>> INSERT INTO todos (description) VALUES ('Do a thing 1');
>>> INSERT INTO todos (description) VALUES ('Do a thing 2');
>>> INSERT INTO todos (description) VALUES ('Do a thing 3');
>>> INSERT INTO todos (description) VALUES ('Do a thing 4');
>>> select * from todos;
Model View Controller (MVC) Heading
Model View Controller (MVC)
ND004 C01 L05 04 Model View Controller (MVC)
Takeaways
- MVC stands for Model-View-Controller, a common pattern for architecting web applications
- Describes the 3 layers of the application we are developing
Layers
- Models manage data and business logic for us. What happens inside models and database, capturing logical relationships and properties across the web app objects
- Views handles display and representation logic . What the user sees (HTML, CSS, JS from the user's perspective)
- Controllers : routes commands to the models and views, containing control logic . Control how commands are sent to models and views, and how models and views wound up interacting with each other.
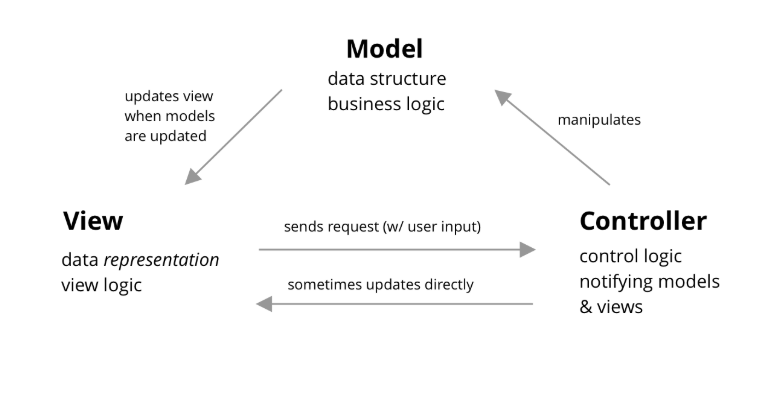
Model-View-Controller Diagram
MVC Quiz
QUIZ QUESTION: :
See if you can match each MVC layer with its description.
ANSWER CHOICES:
Description |
MVC layer |
---|---|
The Models layer |
|
The Views layer |
|
The Controllers layer |
SOLUTION:
Description |
MVC layer |
---|---|
The Models layer |
|
The Views layer |
|
The Controllers layer |
Model View Controller (MVC) Quiz
SOLUTION:
ModelsLayers Quiz
QUIZ QUESTION: :
For each piece of code, match them to what application layer they correspond to
ANSWER CHOICES:
Code |
Layer |
---|---|
Controllers |
|
Views |
|
Models |
|
Data |
SOLUTION:
Code |
Layer |
---|---|
Controllers |
|
Views |
|
Models |